CT 4,5,6 QUESTIONS : DOWNLOAD NOW
CT -4
1. What is inheritance? How does it enable code reusability, explain with
an example?
A.)The capability of a class to derive properties and characteristics from another class is called Inheritance. Inheritance is one of the most important features of Object-Oriented Programming.
- Sub Class: The class that inherits properties from another class is called Sub class or Derived Class.
- Super Class:The class whose properties are inherited by sub class is called Base Class or Super class.
- Reusability: Inheritance supports the concept of “reusability”, i.e. when we want to create a new class and there is already a class that includes some of the code that we want, we can derive our new class from the existing class. By doing this, we are reusing the fields and methods of the existing class.
Syntax:
class subclass_name : access_mode base_class_name { // body of subclass
- Single Inheritance
- Multiple Inheritance
- Hierarchical Inheritance
- Multilevel Inheritance
- Hybrid Inheritance (also known as Virtual Inheritance)
5. Hybrid (Virtual) Inheritance: Hybrid Inheritance is implemented by combining more than one type of inheritance. For example: Combining Hierarchical inheritance and Multiple Inheritance.
Below image shows the combination of hierarchical and multiple inheritance:
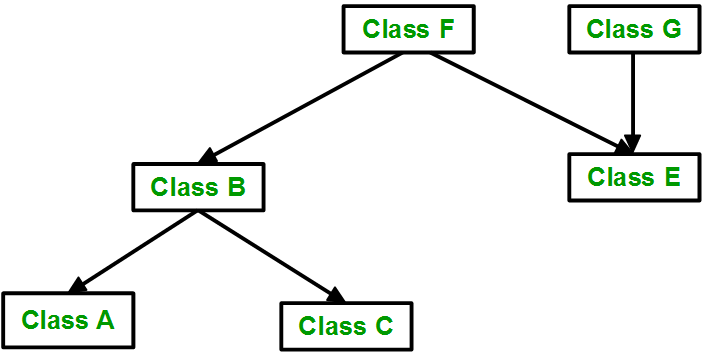
- CPP
OutputThis is a Vehicle
Fare of Vehicle
5. Hybrid (Virtual) Inheritance: Hybrid Inheritance is implemented by combining more than one type of inheritance. For example: Combining Hierarchical inheritance and Multiple Inheritance.
Below image shows the combination of hierarchical and multiple inheritance:
- CPP
This is a Vehicle Fare of Vehicle
Single Inheritance
In this type of inheritance one derived class inherits from only one base class. It is the most simplest form of Inheritance
Syntax:
class subclass_name : access_mode base_class
{
// body of subclass
};
OutputThis is a Vehicle
4. Hierarchical Inheritance: In this type of inheritance, more than one sub class is inherited from a single base class. i.e. more than one derived class is created from a single base class.
OutputThis is a Vehicle
This is a Vehicle
In this type of inheritance one derived class inherits from only one base class. It is the most simplest form of Inheritance
Syntax:
class subclass_name : access_mode base_class { // body of subclass };
This is a Vehicle
4. Hierarchical Inheritance: In this type of inheritance, more than one sub class is inherited from a single base class. i.e. more than one derived class is created from a single base class.
OutputThis is a Vehicle This is a Vehicle
C++ Multilevel Inheritance
In C++ programming, not only you can derive a class from the base class but you can also derive a class from the derived class. This form of inheritance is known as multilevel inheritance.
class A { ... .. ... }; class B: public A { ... .. ... }; class C: public B { ... ... ... };
Here, class B is derived from the base class A and the class C is derived from the derived class B.
Example 1: C++ Multilevel Inheritance
#include <iostream>
using namespace std;
class A {
public:
void display() {
cout<<"Base class content.";
}
};
class B : public A {};
class C : public B {};
int main() {
C obj;
obj.display();
return 0;
}
Output
Base class content.C++ Multiple Inheritance
C++ Multiple Inheritance
In C++ programming, a class can be derived from more than one parent. For example, A class Bat is derived from base classes Mammal and WingedAnimal. It makes sense because bat is a mammal as well as a winged animal.
Example 2: Multiple Inheritance in C++ Programming
#include <iostream>
using namespace std;
class Mammal {
public:
Mammal() {
cout << "Mammals can give direct birth." << endl;
}
};
class WingedAnimal {
public:
WingedAnimal() {
cout << "Winged animal can flap." << endl;
}
};
class Bat: public Mammal, public WingedAnimal {};
int main() {
Bat b1;
return 0;
}
Output
Mammals can give direct birth. Winged animal can flap.
set-5
1a). What are virtual functions? Describe the rules for declaring virtual function?
C++ virtual function
- A C++ virtual function is a member function in the base class that you redefine in a derived class. It is declared using the virtual keyword.
- It is used to tell the compiler to perform dynamic linkage or late binding on the function.
- There is a necessity to use the single pointer to refer to all the objects of the different classes. So, we create the pointer to the base class that refers to all the derived objects. But, when base class pointer contains the address of the derived class object, always executes the base class function. This issue can only be resolved by using the 'virtual' function.
- A 'virtual' is a keyword preceding the normal declaration of a function.
- When the function is made virtual, C++ determines which function is to be invoked at the runtime based on the type of the object pointed by the base class pointer.
Rules of Virtual Function
- Virtual functions must be members of some class.
- Virtual functions cannot be static members.
- They are accessed through object pointers.
- They can be a friend of another class.
- A virtual function must be defined in the base class, even though it is not used.
- The prototypes of a virtual function of the base class and all the derived classes must be identical. If the two functions with the same name but different prototypes, C++ will consider them as the overloaded functions.
- We cannot have a virtual constructor, but we can have a virtual destructor
Output:
Derived Class is invoked
1 b) Explain pointer to a class in C++ with an example program?
A pointer to a C++ class is done exactly the same way as a pointer to a structure and to access members of a pointer to a class you use the member access operator -> operator, just as you do with pointers to structures. Also as with all pointers, you must initialize the pointer before using it.
#include <iostream> using namespace std; class Box { public: // Constructor definition Box(double l = 2.0, double b = 2.0, double h = 2.0) { cout <<"Constructor called." << endl; length = l; breadth = b; height = h; } double Volume() { return length * breadth * height; } private: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }; int main(void) { Box Box1(3.3, 1.2, 1.5); // Declare box1 Box Box2(8.5, 6.0, 2.0); // Declare box2 Box *ptrBox; // Declare pointer to a class. // Save the address of first object ptrBox = &Box1; // Now try to access a member using member access operator cout << "Volume of Box1: " << ptrBox->Volume() << endl; // Save the address of second object ptrBox = &Box2; // Now try to access a member using member access operator cout << "Volume of Box2: " << ptrBox->Volume() << endl; return 0; }
output:
Constructor called. Constructor called. Volume of Box1: 5.94 Volume of Box2: 102
2.Explain about dynamic binding with an example program?
The binding which can be resolved by the compiler using runtime is known as static binding. For example, all the final, static, and private methods are bound at run time. All the overloaded methods are binded using static binding.
The concept of dynamic binding removed the problems of static binding.
Dynamic binding
The general meaning of binding is linking something to a thing. Here linking of objects is done. In a programming sense, we can describe binding as linking function definition with the function call.
So the term dynamic binding means to select a particular function to run until the runtime. Based on the type of object, the respective function will be called.
As dynamic binding provides flexibility, it avoids the problem of static binding as it happened at compile time and thus linked the function call with the function definition.
Use of dynamic binding
On that note, dynamic binding also helps us to handle different objects using a single function name. It also reduces the complexity and helps the developer to debug the code and errors.
How to implement dynamic binding?
The concept of dynamic programming is implemented with virtual functions.
Virtual functions
A function declared in the base class and overridden(redefined) in the child class is called a virtual function. When we refer derived class object using a pointer or reference to the base, we can call a virtual function for that object and execute the derived class's version of the function.
3 a). a) Explain this pointer with an example program?
Every object in C++ has access to its own address through an important pointer called this pointer. The this pointer is an implicit parameter to all member functions. Therefore, inside a member function, this may be used to refer to the invoking object.
Friend functions do not have a this pointer, because friends are not members of a class. Only member functions have a this pointer.
#include <iostream> using namespace std; class Box { public: // Constructor definition Box(double l = 2.0, double b = 2.0, double h = 2.0) { cout <<"Constructor called." << endl; length = l; breadth = b; height = h; } double Volume() { return length * breadth * height; } int compare(Box box) { return this->Volume() > box.Volume(); } private: double length; // Length of a box double breadth; // Breadth of a box double height; // Height of a box }; int main(void) { Box Box1(3.3, 1.2, 1.5); // Declare box1 Box Box2(8.5, 6.0, 2.0); // Declare box2 if(Box1.compare(Box2)) { cout << "Box2 is smaller than Box1" <<endl; } else { cout << "Box2 is equal to or larger than Box1" <<endl; } return 0; }
output:
Constructor called. Constructor called. Box2 is equal to or larger than Box1
3b) What is a pointer? Explain features of pointers?
Pointers store address of variables or a memory location.
Syntax:
datatype *var_name;
Example: pointer “ptr” holds address of an integer variable or holds address of a memory whose value(s) can be accessed as integer values through “ptr”
Features of Pointers:
- Pointers save memory space.
- Execution time with pointers is faster because data are manipulated with the address, that is, direct access to
memory location. - Memory is accessed efficiently with the pointers. The pointer assigns and releases the memory as well. Hence it can be said the Memory of pointers is dynamically allocated.
- Pointers are used with data structures. They are useful for representing two-dimensional and multi-dimensional
arrays. - An array, of any type can be accessed with the help of pointers, without considering its subscript range.
- Pointers are used for file handling.
- Pointers are used to allocate memory dynamically.
try: represents a block of code that can throw an exception.
catch: represents a block of code that is executed when a particular exception is thrown.
throw: Used to throw an exception. Also used to list the exceptions that a function throws, but doesn’t handle itself.
main advantages of exception handling over traditional error handling.
1) Separation of Error Handling code from Normal Code: In traditional error handling codes, there are always if else conditions to handle errors. These conditions and the code to handle errors get mixed up with the normal flow. This makes the code less readable and maintainable. With try catch blocks, the code for error handling becomes separate from the normal flow.
2) Functions/Methods can handle any exceptions they choose: A function can throw many exceptions, but may choose to handle some of them. The other exceptions which are thrown, but not caught can be handled by caller. If the caller chooses not to catch them, then the exceptions are handled by caller of the caller.
In C++, a function can specify the exceptions that it throws using the throw keyword. The caller of this function must handle the exception in some way (either by specifying it again or catching it)
3) Grouping of Error Types: In C++, both basic types and objects can be thrown as exception. We can create a hierarchy of exception objects, group exceptions in namespaces or classes, categorize them according to types.
Output:
Before try Inside try Exception Caught After catch (Will be executed)
2.What are containers? Explain different types of containers in C++?
Containers are the objects used to store multiple elements of the same type or different.
The container library categorizes containers into four types:
- Sequence containers
- Sequence container adapters
- Associative containers
- Unordered associative containers
Let’s dive into each of these categories.
Sequence Containers
Sequence containers are used for data structures that store objects of the same type in a linear manner.
The STL SequenceContainer
types are:
array
represents a static contiguous arrayvector
represents a dynamic contiguous arrayforward_list
represents a singly-linked listlist
represents a doubly-linked listdeque
represents a double-ended queue, where elements can be added to the front or back of the queue.
While std::string
is not included in most container lists, it does in fact meet the requirements of a SequenceContainer
.
Container Adapters
Container adapters are a special type of container class. They are not full container classes on their own, but wrappers around other container types (such as a vector
, deque
, or list
). These container adapters encapsulate the underlying container type and limit the user interfaces accordingly.
Let’s consider std::stack
. std::stack
is a container that enforces a LIFO-type data structure. Here’s the declaration of std::stack
:
Notice that Container
defaults to wrapping a std::deque<T>
. You can actually change the type of underlying container to another STL SequenceContainer
or your own custom container. The container you specify has to meet the following requirements:
- The container must satisfy the requirements of a SequenceContainer
- Must provide
back()
,push_back()
, andpop_back()
interfaces
The STL containers std::vector
, std::deque
, and std::list
all meet these requirements and can be used for underlying storage.
The standard container adapters are:
stack
provides an LIFO data structurequeue
provides a FIFO data structurepriority_queue
provides a priority queue, which allows for constant-time lookup of the largest element (by default)
Associative Containers
Associative containers provide sorted data structures that provide a fast lookup (O(log n) time) using keys.
The STL AssociativeContainer
types are can be divided in two ways: containers which require unique keys, and those which allow multiple entries using the same key.
- Keys are unique
set
is a collection of unique keys, sorted by keysmap
is a collection of key-value pairs, sorted by keysset
andmap
are typically implemented using red-black trees
- Multiple entries for the same key are permitted
Each of the associative containers can specify a comparison function during declaration. Let’s take a look at the definition of std::set
:
The default comparison function for associative containers is std::less
. This comparison function is used to sort keys. If you prefer a different sorting or allocation scheme, you should override these functions during declaration.
Unordered Associative Containers
Unordered associative containers provide unsorted data structures that can be accessed using a hash. Access times are O(n) in the worst-case, but much faster than linear time for most operations.
For all STL UnorderedAssociativeContainer
types, a hashed key is used to access the data. Similar to the AssociativeContainer
, the standard types are split between those that require unique keys, and those that do not:
- Keys are unique
unordered set
is a collection of keys, hashed by keysunordered_map
is a collection of key-value pairs, hashed by keys
- Multiple entires for the same key are permitted
unordered_multiset
is a collection of keys, hashed by keysunordered_multimap
is a collection of key-value pairs, hashed by keys
Like with the other container types, UnorderedAssociativeContainer
types can have details overridden. Let’s look at std::unordered_set
:
You can override the hashing function, the key comparison function, and the allocator. I don’t care to improve up on the STL hashing functionality, so I usually leave these details alone. However, if you want to override any of the default functions, you must do it during declaration.
3. Write a C++ program illustrating bubble sort using function templates?
#include<conio.h>
#include<iostream.h>
//Declaration of template class bubble
template<class bubble>
void bubble(bubble a[], int n)
{
int i, j;
//bubble sort algorithm inside bubble template
for(i=0;i<n-1;i++)
{
for(j=i+1;j<n;j++)
{
if(a[i]>a[j])
{
bubble b;
b=a[i];
a[i]=a[j];
a[j]=b;
}
}
}
}
void main()
{
int arr[20],m,k,i;
char ch[20];
//clrscr();
//Taking values from the user
cout<<"Enter what type of elements you want to sort :\n1. int\n2. char\n";
cin>>m;
if(m==1)
{
cout<<"\nEnter the number of elements you want to enter:";
cin>>k;
cout<<"\nEnter elements:";
for(i=0;i<k;i++)
cin>>arr[i];
//call to bubble for inter sorting
bubble(arr,6);
cout<<"\nSorted Order Integers: ";
for(i=0;i<k;i++)
cout<<arr[i]<<"\t";
}
else
{
cout<<"\nEnter the number of characters you want to enter:";
cin>>k;
cout<<"\nEnter elements:";
for(i=0;i<k;i++)
cin>>ch[i];
//call to bubble for character sorting
bubble(ch,4);
cout<<"\nSorted Order Characters: ";
for(i=0;i<k;i++)
cout<<ch[i]<<"\t";
}
getch();
}
Information from Ravikanh Sir,
All need to submit OOP C++ Classtests 04,05 and 06 by answering questions in ct papers and submit the written answer scripts by monday i.e. 31-01-2022(monday) 02:00PM
If any body unable to attend college can send writen answer scripts (HARDCOPY) by other students.
If any failed to submit wihin time will be considered as ABSENT for the assessement.
Instructions:
1.Write and submit only on c papers.
2. Clearly write all details like rollno, ct number, date(given in question paper).